Fragment是依赖于Activity的,不能独立存在的。Android运行在各种各样的设备中,有小屏幕的手机,还有大屏幕的平板,电视等。同样的界面在手机上显示可能很好看,在大屏幕的平板上就未必了,手机的界面放在平板上可能会有过分被拉长、控件间距过大等情况。针对屏幕尺寸的差距,Fragment的出现能做到一个App可以同时适应手机和平板。(后续会更新)
fragment生命周期
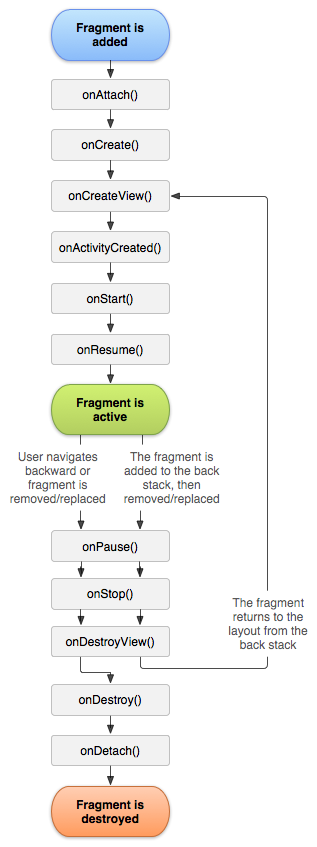
Fragment 的生命周期和 Activity 很相似,只是多了一下几个方法:
方法名 | 说明 |
onAttach() | 在Fragment 和 Activity 建立关联是调用(Activity 传递到此方法内)。 |
onCreateView() | 当Fragment 创建视图时调用。 |
onActivityCreated() | 在相关联的 Activity 的 onCreate() 方法已返回时调用。 |
onDestroyView() | 当Fragment中的视图被移除时调用。 |
onDetach() | 当Fragment 和 Activity 取消关联时调用。 |
可以看下几种操作情况下Fragment 的生命周期变化
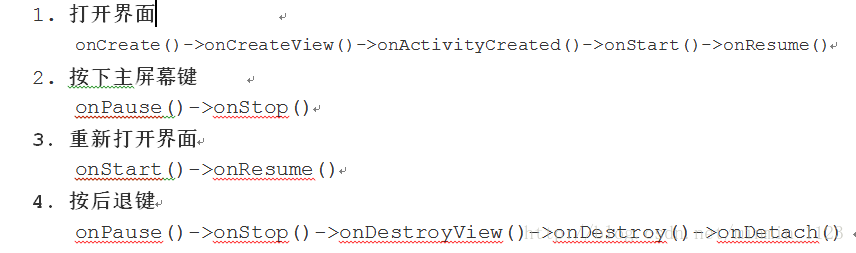
管理 Fragment 生命周期和 Activity 生命周期很相似,同时 Activity 的生命周期对 Fragment 的生命周期也有一定的影响,如下图所示
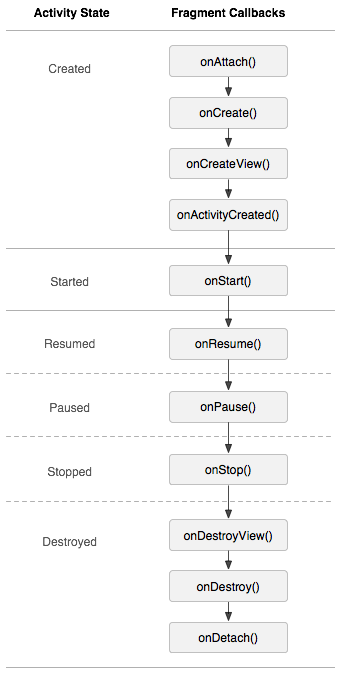
用下图(来源)来表示 Activity 和 Fragment 的生命周期变化的先后过程是:
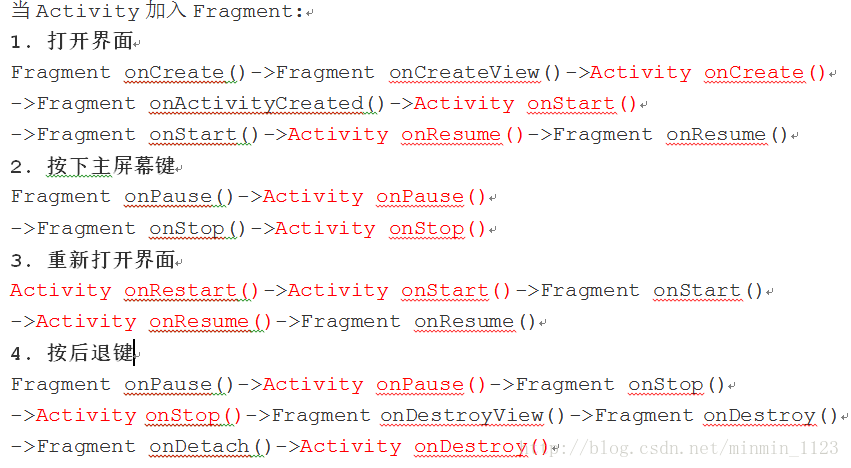
.
fragment静态调用
在 Activity 的布局文件内声明片段
1、新建一个Layout的xml文件,什么组件都行,我是往里面放了个TextView,取名为layout_textview
2、新建一个Java类,继承fragment,重写onCreateView方法。我这里取名为TextViewFragment(需要导入androidx.fragment.app.Fragment)
package com.omniscience.fragment;
import android.os.Bundle;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import androidx.annotation.NonNull;
import androidx.annotation.Nullable;
import androidx.fragment.app.Fragment;
public class TextViewFragment extends Fragment{
@Nullable
@Override
public View onCreateView(@NonNull LayoutInflater inflater, @Nullable ViewGroup container, @Nullable Bundle savedInstanceState) {
return inflater.inflate(R.layout.layout_textview, container, false);
}//inflater.inflate()的第一个参数写上上一步xml文件的文件名
}
inflater.inflate():其实就是LayoutInflater.inflate(),这个函数而言,功能是将一段 XML 资源文件加载成为 View。所以通常用于将 XML 文件实例化为 View。然后获取 View 上的组件最后操作之。
关于LayoutInflater.inflate(),详细可以看这个博客:探索 Android Inflater.inflate()
onCreateView:Fragment的生命周期中的一种状态,在为碎片创建视图(加载布局)时调用,这个方法返回View对象。
onCreateView的属性:
LayoutInflater inflater:作用类似于findViewById(),findViewById()用来寻找xml布局下的具体的控件(Button、TextView等),LayoutInflater inflater()用来找res/layout/下的xml布局文件
ViewGroup container:表示容器,View放在里面
Bundle savedInstanceState:保存当前的状态,在活动的生命周期中,只要离开了可见阶段,活动很可能就会被进程终止,这种机制能保存当时的状态
3、最后在xml里加入fragment标签
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<fragment
android:id="@+id/text_fragment"
<!--name属性的属性值为java类的名字-->
android:name="com.omniscience.fragment.TextViewFragment"
android:layout_width="wrap_content"
android:layout_height="wrap_content">
</fragment>
</LinearLayout>
其中 fragment 中的 android:name 属性要指定 fragment 对应的具体包名路径,当系统创建此 Activity 布局时,会实例化在布局中指定的每个 fragment,并为每个 fragment 调用 onCreateView()方法,以检索每个 fragment 的布局。系统会直接插入 fragment 返回的 View 来替代 fragment 元素。
并且在 Activity 活动里可以直接使用 findViewById() 方法获取 fragment 对应布局里的控件。同样在 fragment 里可以直接使用 getActivity()方法获得绑定的主 Activity 实例,并调用 Activity 里的方法或其他 fragment 实例。
.
fragment动态添加
通过编程方式将 fragment 添加到某个activity布局里现有的 ViewGroup (例如 LinearLayout 或 FrameLayout)里
要想在 Avtivity 中执行 Fragment 事务 (如添加、删除或替换 Fragment),必须使用 FragmentTransaction 中的 API。可以使用下面这样从 Activity 中获取一个 FragmentTransaction。
FragmentManager fragmentManager = getFragmentManager();
FragmentTransaction fragmentTransaction = fragmentManager.beginTransaction();
然后可以使用 add()方法添加一个 fragment ,指定要添加的 fragment 和插入到哪个视图。
ExampleFragment exampleFragment = new ExampleFragment();
fragmentTransaction.add(R.id.frame_layout,exampleFragment);
fragmentTransaction.commit();
add 方法中第一个参数是一个activity 对应布局文件中的 ViewGroup,即应该放置 fragment 的位置,由资源 ID 指定,第二个参数是加入的 fragment ,一旦通过 fragmentTransaction 做了更改,最后必须使用 commit 方法以使更改生效。
注意:如果Android系统为3.0以下,那么就要使用getSupportFragmentManager()
例如:
package com.omniscience.fragment;
import androidx.appcompat.app.AppCompatActivity;
import androidx.fragment.app.Fragment;
import androidx.fragment.app.FragmentManager;
import androidx.fragment.app.FragmentTransaction;
import android.os.Bundle;
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
FragmentManager fragmentManager = getSupportFragmentManager();
FragmentTransaction fragmentTransaction = fragmentManager.beginTransaction();
fragmentTransaction.add(R.id.listFragment, new ListViewFragment());
fragmentTransaction.commitNow();
}
}
.
fragment动态删除
与上述类型相似,fragmentTransaction.remove(fragment)
Comments | NOTHING